Some useful Linux snippets
Some useful Linux commands and scripts
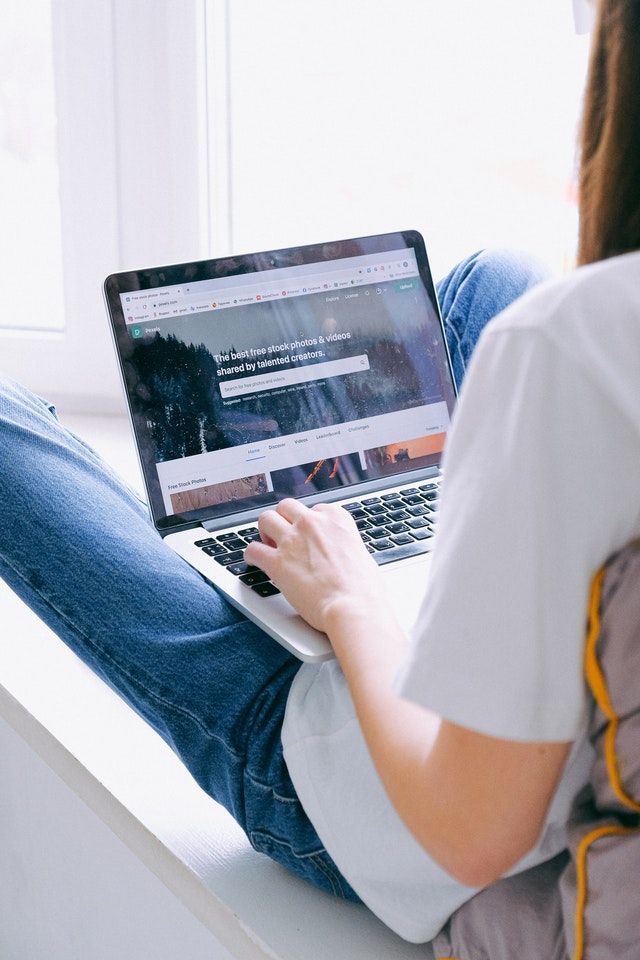
list installed software
apt list --installed
install from .deb
file
sudo dpkg -i <n.deb>
configure firewall
# block all incoming
# allow all outgoing
# all/limit common ports
# enable ufw
# show ufw status
ufw default deny incoming
ufw default allow outgoing
ufw limit 22/tcp
ufw allow 80/tcp
ufw allow 443/tcp
ufw enable
ufw status verbose
install fail2ban
sudo apt install fail2ban
sudo systemctl enable fail2ban
sudo systemctl start fail2ban
check listening ports
netstat -tunlp
move folder between users
mv /root/ghost ~vmadmin/
folder sizes
# disk usage with sizes but go to a depth of 1 and sort
# this means i just want the cumulative value and not individual sizes per object recursively down through the folder hierarchy
du -h -d 1 | sort -h
install from url
# install mockoon
wget https://github.com/mockoon/mockoon/releases/download/v1.11.0/mockoon-1.11.0.deb -O kam.deb && sudo dpkg -i kam.deb
How to create a swapfile
Resolution
-
allocate the size
sudo fallocate -l 1G /swapfile
-
zero out the file (takes about a minute)
sudo dd if=/dev/zero of=/swapfile bs=1024 count=1048576
-
make the file only accessible by
root
sudo chmod 600 /swapfile
-
create the swapfile
sudo mkswap /swapfile
-
turn the swapfile on
sudo swapon /swapfile
-
register the changes with the system
sudo vi /etc/fstab
(append line)/swapfile swap swap default 0 0
-
validate the configuration with the file system
sudo mount -a
How to display mapped drives
Resolution
Use the display filesystem
command:
df -aTh
Constructing json
export SAMPLE_CONTAINER=test
export SAMPLE_FILE=test.jar
export SAMPLE_TARGET=/opt/test/test.jar
echo $( jq -n \
--arg container "$SAMPLE_CONTAINER" \
--arg file "$SAMPLE_FILE" \
--arg target "$SAMPLE_TARGET" \
'{mycontainer: $container, myfile: $file, mytarget: $target}' )
{ "mycontainer": "test", "myfile": "test.jar", "mytarget": "/opt/test/test.jar" }
If statement on function, disecting json return to determine if value exists
if [[ $(az rest --method GET --uri https://graph.microsoft.com/v1.0/servicePrincipals/5cf3c8e1-4e18-4114-9e4d-8c86577fd17f/appRoleAssignedTo -o json | jq '[.value[] | select(.principalId == "bada8227-4452-451d-88d5-647b669cea07")] | length'==0 | grep 'true') = 'true' ]]; then echo 'not here!'; fi
Get value from json snippet in file where attribute is something
projectID=$(jq -r --arg EMAILID "$EMAILID" '
.resource[]
| select(.username==$EMAILID)
| .id' file.json)
Create an alias with parameters
alias kam='function (){ls $1 && printenv | grep -i $2}'
Running a call: kam system arm
will list the folder "system" and grep for environment variables containing the word "arm"
Get got branch name from bash
# get git branch name
git branch 2> /dev/null | sed -e '/^[^*]/d' -e 's/* \(.*\)/ (\1)/'
Creating named parameters on a bash script
#!/bin/bash
# allows for named params on the cli eg. myscript --param1 true --param2 58
myapi=${myapi:-false}
myapi2=${myapi2:-false}
myapi3=${myapi3:-false}
while [ $# -gt 0 ]; do
if [[ $1 == *"--"* ]]; then
param="${1/--/}"
declare $param="$2"
# echo $1 $2 # Optional to see the parameter:value result
fi
shift
done
if [ "$myapi" = "true" ]; then ./myapi/myapi_tests.sh; fi
if [ "$myapi" = "false" ] &&
[ "$myapi2" = "false" ] &&
[ "$myapi3" = "false" ];
then echo "No tests were set to run"; fi
Set the current directory to be the working directory (useful in scripts)
# set working directory - useful in scripts where you want to call from somewhere else but the script usws relative paths
cd "$(dirname "$0")"
Find the last return code
# find last return code
if [ $? -eq 0 ]; then echo 'ok!'; else echo 'no ok!'; fi
Retry loop
max_retry_count=3
retry=1
seconds_to_sleep=5
sleep_increment=5
mycommand=" \
az vm show -g vm-test \
-n vm-test \
"
until [ $retry -gt $max_retry_count ] || eval $mycommand
do
sleep $seconds_to_sleep
((sleeper=seconds_to_sleep+$sleep_increment))
echo "Attempt ($retry)"
((retry++))
done
Upper and lowercase
firstname=KAM
lastname=lagan
echo "${firstname:l} ${lastname:u}"
outputs
kam LAGAN
Hide the output of a command
command > /dev/null 2>&1
Explanation:
command > /dev/null
redirects the output of command (stdout) to/dev/null
2>&1
redirects stderr to stdout, so errors (if any) also goes to/dev/null
Keyboard produces ABCD
characters when using arrow keys in vi
The three operations I found that helped me:
- Create a
~/.vimrc
file - Add
set nocompatible
to the file - Install vim:
sudo apt-get install vim
Now restart your terminal and things should hopefully work out for ya!